Vue3入门
前言
Vue3.0.0已经发布了,是时候迎头赶上了,视频教程也已经有了。 关于Vue3.0的重大的改进,反正我觉得和Vue2.0还是有不小的变动的,特别的就是这个setup函数。我自己感觉水平不够,看不出来有什么好的地方,写法不同了,思想不同了,性能不同了。
(1) 主要就是computed计算属性我觉得有点不好用了,进行计算属性就必须写.value,不好用,而且配合vuex的时候,可能不需要响应式数据,但是最后还是会变成响应式的
(2) 好用的地方,就是单文件组件不需要必须有一个根div了
(3) 还有就是代码组织我感觉容易乱吧,vuex我觉得也有点控制不住了,特别是对于对象的引用,比如本来只是作为数据存储的一个对象,不需要进行响应式,但是放到vuex中,就变成了响应式了,需要使用shallow这种语法吧。
1.不要再用 Vue 2 的思维写 Vue 3 了
2.Vue3.2 setup语法糖、Composition API归纳总结 这个其实也挺好的,其中常用到的一些操作都有说明
3.vue3 watch不生效_让你30分钟了解vue 3 一个完成的vue 3.x 完整组件模版结构包含了:组件名称、 props、components、setup(hooks、computed、watch、methods 等)
4.Vue 中的 defineComponent 介绍了为什么在写组件的时候,不用 export default {},而变成了 export default defineComponent({}),主要就是为了方便编译器进行类型推导用的。
1.创建项目
1 | ## 安装 |
创建项目的时候,最新的vue-cli有一个选项就是选择vue的版本。
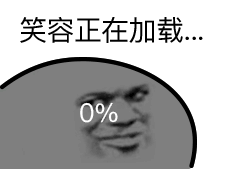
1.vue cli 3.0快速创建项目 (这里是vue cli3.0而不是vue3.0创建的项目)
2.Vue3 “export ‘createWebHistory, createRouter‘ was not found in ‘vue-router‘
3.Vue-Cli 创建vue3项目
2.vue-router
(1) 安装
1 | yarn add vue-router@next --save |
(2) 编写
1 | import { |
(3) 引用 router-view
这里可以直接使用 router-view 了,不需要外层再包裹一层 div
1 | <template> |
(4) 注入
1 | import { createApp } from 'vue' |
(5) 获取父路由
1 | // 获取路由记录 |
1.vue3.0 集成vue-router、vuex
2.Vue3.0迁移(Vue Router篇)
3.vue3.0配置路由导航守卫和路由元信息 一个进行页面登陆和授权的导航路由实例,加一个元信息,然后我们在router.beforeEach上读取这个信息
4.vue获取父路由 在钩子函数中获取路由
5.vue3.0路由route/router的使用
6.路由的使用
4.vuex
(1) 安装
1 | ## yarn |
5.配置路径别名
1 | const path = require('path') |
1.vue3.0以后添加路径别名
6.动态组件
在使用3.0的使用,如果引入动态组件,像下面这样,就会报错
1 | export default { |
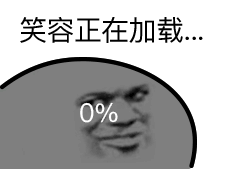
【解决方法】
使用新的 defineAsyncComponent 定义组件。
1 | import { defineAsyncComponent } from 'vue' |
7.响应式数组
响应式数据有一个问题,如果你将一个对象变成了响应式的,如果改变了整个对象的指向,那么这个还是响应式的吗?这个问题留着我去问别人。
如果你生命了一个数组,不能通过改变对象的值进行响应,错误的示例
1 | let legend=reactive([ |
正确的示例:
【方法一】
1 | // 直接使用ref |
【方法二】
1 | // 直接使用reactive |
1.Vue3.0(四)ref源码分析与toRefs
2.vue3使用reactive包裹数组如何正确赋值
3.vue3使用reactive包裹数组如何正确赋值
4.Vue3 Composition API: 对比ref和reactive ref 的作用就是将一个原始数据类型(primitive data type)转换成一个带有响应式特性;reactive (等价于Vue2中的Vue.observable() )来赋予对象(Object) 响应式的特性;响应型对象(reactive object) 转化为普通对象(plain object),同时又把该对象中的每一个属性转化成对应的响应式属性(ref);
5.vue3获取原始对象方法,禁止响应式toRaw
6.vue3.0的toRaw和markRaw toRaw 返回 reactive 或 readonly 代理的原始对象。这是一个转义口,可用于临时读取而不会引起代理访问/跟踪开销,也可用于写入而不会触发更改
7.vue3学习–readonly与shallowReadonly、toRaw与markRaw、toRef的特点与作用
8.vue3.0数组清空与重新赋值 数组用ref([])来声明,然后用list.value = []来修改
9.Vue3实践指南:使用reactive函数声明数组如何正确赋值响应式、script setup语法糖中toRefs如何优雅呈现、Options API 与 Composition API 如何选择及混用是否对性能有影响、关于 setup 中没有 this 的问题及 setup 的执行时机
8.props
【方法一】
使用 props 属性进行内容的传递
父组件
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21<template>
<child :attr="curr">
</template>
<script>
import child from './child';
import {reactive} from "vue";
export default {
setup(){
const curr=reactive({
value:{}
}); // 当前选中对象
return {
curr
}
}
}子组件
在使用的时候,我发现如果不单独的定义这个 props ,在 setup 里面就会得到一个 undifined 的值。1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25import {toRefs,computed} from "vue";
export default {
props: {
attrs: {
require:true,
type:Object,
}
},
setup(props){
/**
* 数据部分
*/
const currFeature=toRefs(props.attrs.value);
/** 计算属性 */
const currInfo = computed(() => {
return currFeature?currFeature.properties:{};
});
return {
currInfo
}
}
}
1.vue3组合式api中的ref、toRef、toRefs三个函数的简单使用示例
2.vue3 setup使用props
3.Props 这是官方的解释,我发现现在越来越多的就是使用 setup 这种单文件的说明了。
9.watch
(1) 像下面的例子中,watch监听到了props的变化,但是无法更新页面上的currInfo和currProps的值,不知道为什么啊。
1 | setup(props){ |
1.vue中watch不触发、不生效的解决办法及原理
2.vue3 使用watch监听数组问题
3.Vue3第一篇之ref和reactive详解扩展
4.vue3讲解setup,ref,reactive和watch语法
(2) 无法进行深度监听
我在vuex中有一个对象
1 | const store = createStore({ |
当我在一个组件中,改变这个值的时候
1 | const yujing_info=computed(()=>store.state.yujing_info); |
我期望的在另外的一个组件中的监听函数
1 | // setup中的监测 |
但是监听失败了,当在第二步,改变transed 值当时候,第三步的监听函数并不会执行
【解决方法】
后来去掉了computed的监听,直接修改了store.state上的属性,这个时候,即便是注释掉了 commit, 也能实现响应式监听。
1 | // 更新转移人数 |
1.为什么vue3 watch( )无法监听对象属性
2.Vue3.0 | reactive/effect/computed
3.Vue3.0性能优化之toRaw,markRaw
4.vue3中reactive和shallowRef的区别
5.vue3.0 watch监听对象不生效
10.事件监听
使用事件的时候,出现了问题:Extraneous non-emits event listeners (update, canel) were passed to component but could not be automatically inherited because component renders fragment or text root nodes. If the listener is intended to be a component custom event listener only, declare it using the “emits” option.
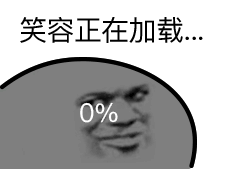
【解决方法】
就是在子组件中声明需要跑出的事件列表
1 | export default { |
1.javascript - vue 3发出警告 “Extraneous non-emits event listeners”
2.vue3.0版本中怎么使用emit向父组件传值(TS)
3.Vue3 declare it using the “emits” option警告 需要在emits中定义抛出的事件名
11.异步组件相互依赖
两个都是异步组件,但是一个组件依赖于另外一个组件,这个时候怎么办呢?