Nodejs之Restify
使用nodejs开发restful风格的api,我先选中了restify。其实为什么选择这个,而不是experss,这就因人而异了。其实对于一个架构师来说(我虽然没做过),如何再各种技术中取舍,也是一门学问,是激进的使用更新的技术,还是沿用团队成员都在使用的老的方法。要知道,任何一种架构的选择,以及技术的变更,都是需要成本的。再用新技术换来时间和空间成本的同时,也要照顾着自己以及其他人的学习成本。所以,开始任何一项技术研究之前,要做的就是对比各种技术的优缺点,要知道,任何一种技术和语言都不是完美的,都有其适用的场景。下面几篇文章,是restify和express的比较。
我觉得可能有两种不同,如果有界面,用express,如果只是用来做api,用restify。
1.求对比 restify 和 express:https://cnodejs.org/topic/543cc9fc91eadb0f73aa3498
2.Comparing Express, Restify, hapi and LoopBack for building RESTful APIs:https://strongloop.com/strongblog/compare-express-restify-hapi-loopback/
1.安装
cnpm install restify -D
2.获取post请求参数
要使用use对请求进行拦截,然后用plugins.bodyParser解析body的内容,否则就获取不到body中的内容。
1 | var restify = require('restify'); |
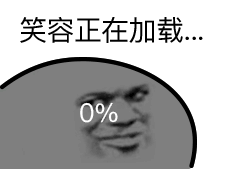
3.设置跨域访问
1 | server.use(function(req,res,next){ |
pre:在确定路由之前执行的处理器链。
use:在确定路由之后执行的处理器链。
1.restify 中文手册:https://xiie.github.io/2016/10/restify-chinese-manual/
2.Node.js express 跨域问题:https://cnodejs.org/topic/51dccb43d44cbfa3042752c8
4.全局异常
像后台语言,比如Java、Php做Api开发,即使函数里面出现了错误,整个程序还是继续运行的,但是像nodejs这种,你要是写个没有捕获的异常,程序直接就退出了。比如我这里的JSON.parse(body),如果传入的参数中没有这个值,就会直接报错退出nodejs。
比如我这里的:
1 | server.post('/api/kriging', function (req, res, next) { |
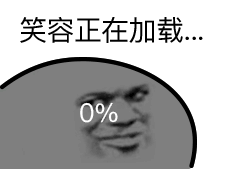
当然你可以在每个方法里面,都用try{}catch{} 去捕获异常,可是这似乎不是长久之计。
我尝试了使用nodejs的全局异常处理方式
1 | process.on("uncaughtExpection",function(){ |
都不起作用。
刚开始我也添加了很多的restifyError,依然不起作用。
1 |
|
最后解决方式是,再参考五中,找到了答案。在创建server对象时,添加 handleUncaughtExceptions: true 参数。这样,上面的server.on(“uncaughtException”) 就起作用了。
1 | const server = restify.createServer({ |
1.Express中全局异常处理:https://segmentfault.com/q/1010000009295251
2.NodeJS和Express下的全局异常捕获处理:http://www.haiyang.me/read.php?key=765
3.在Express和Restify中处理uncaughtException:http://cn.voidcc.com/question/p-nyxspzqs-bcp.html
4.Express 框架中对错误的统一处理:https://itbilu.com/nodejs/npm/41ctyLryW.html
5.https://github.com/restify/node-restify/issues/1749