Openlayers结合Geoserver实现修改元素
先来点铺垫,主要查询下有哪些东西,什么是WFS-T服务。
1.WFS reference:https://docs.geoserver.org/latest/en/user/services/wfs/reference.html
2.Modifying Feature Types:https://geoserver.geo-solutions.it/edu/en/vector_data/wfst.html
3.Getting Transaction Support enabled in WFS on GeoServer (Boundless OpenGeoSuite)?:https://gis.stackexchange.com/questions/141031/getting-transaction-support-enabled-in-wfs-on-geoserver-boundless-opengeosuite
4.通过wfs修改要素:http://weilin.me/ol3-primer/ch12/12-01-04.html (扯淡大叔,这个步骤讲到的非常的全面)
5.最近在学习OpenLayer教程,通过OpenLayer加载geoserver中图层,其中遇到的问题并解决在此做下记录:https://blog.csdn.net/wo_buzhidao/article/details/79268902
直接上代码:
1 |
|
其中添加天地图的代码就不在这里写了,主要思路就是添加底图,添加select,添加modifyInteraction,然后监听modifyend函数,最后在保存的时候,将修改的图形使用writeTransaction序列化为xml。示例xml如下:
1 | <Transaction xmlns="http://www.opengis.net/wfs" service="WFS" version="1.1.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.opengis.net/wfs http://schemas.opengis.net/wfs/1.1.0/wfs.xsd"><Update typeName="feature:pipeline" xmlns:feature="proheng"><Property><Name>geometry</Name><Value><MultiLineString xmlns="http://www.opengis.net/gml" srsName="EPSG:4326"><lineStringMember><LineString srsName="EPSG:4326"><posList srsDimension="2">120.34757794 30.33886672 120.34854859113693 30.339445173740387 120.34748333 30.34026191</posList></LineString></lineStringMember></MultiLineString></Value></Property><Property><Name>FID_</Name><Value>0</Value></Property><Property><Name>LineNumber</Name><Value>1</Value></Property><Property><Name>EndLat</Name><Value>120.347483332</Value></Property><Property><Name>EndLon</Name><Value>30.3402619111</Value></Property><Property><Name>StartLon</Name><Value>30.3388667219</Value></Property><Property><Name>StartLat</Name><Value>120.347577944</Value></Property><Filter xmlns="http://www.opengis.net/ogc"><FeatureId fid="pipeline.18"/></Filter></Update></Transaction> |
最后更新成功,返回的内容:
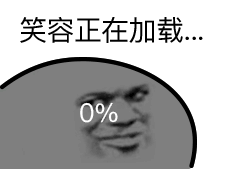
图形修改成功:
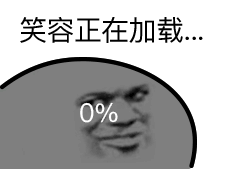
问题
(1) Feature type ‘pipeline’ is not available
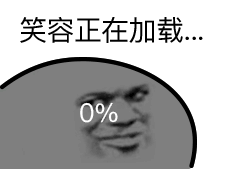
主要是因为在写featureNS时写错了
1 | var featObject = WFSTSerializer.writeTransaction(null, |
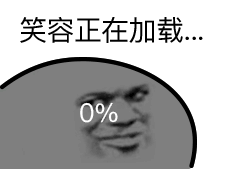
其中的featureNS是工作空间的URI
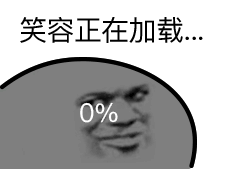
其中的featureType,是图层的名字,不带工作空间
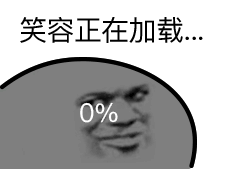
1.Geoserver WFS-T “Feature type ‘Workspace:Layer’ is not available” Error on update / delete:https://gis.stackexchange.com/questions/172112/geoserver-wfs-t-feature-type-workspacelayer-is-not-available-error-on-updat
(2) No such property: geometry
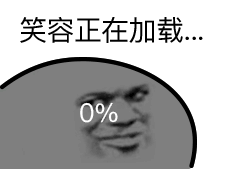
尝试解决方式是将xml中的feature:myLayer,改为myNameSpace:myLayer
1 | var str=new XMLSerializer().serializeToString(node); |
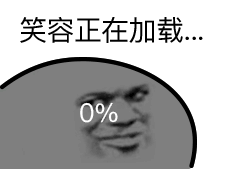
这中方式是不对的。
实际上,查看这个xml,其中的图形属性是geometry
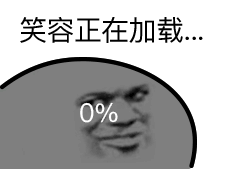
但是实际上图形属性的名称是the_geom而不是geometry
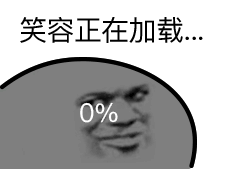
应该将其中的xml中的gemtory改为the_geom
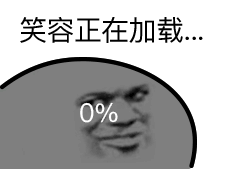
如果是使用postgis发布的底图,那么这个图形属性的名称可能为geom,根据具体情况进行调整
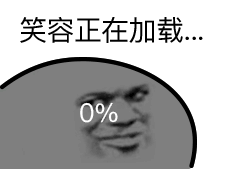
1.No such property: geometry when updating feature in openlayers 3 - Geoserver:https://stackoverflow.com/questions/37250892/no-such-property-geometry-when-updating-feature-in-openlayers-3-geoserver
(3) {proheng}pipeline is read-only(这里的proheng和pipeline不需要知道具体含义,就是工作空间和图层的名字))
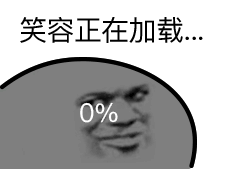
解决方式主要是打开geoserver的控制面板,左侧的Security->Data
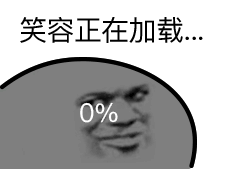
然后点击“*.*.w”,这一栏,然后选择:Grant access to any role
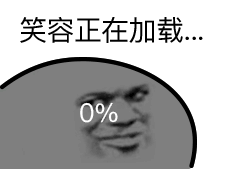
重启geoserver
1.geoserver wfs-t is read-only exception:https://stackoverflow.com/questions/41972613/geoserver-wfs-t-is-read-only-exception
2.最近在学习OpenLayer教程,通过OpenLayer加载geoserver中图层,其中遇到的问题并解决在此做下记录:https://blog.csdn.net/wo_buzhidao/article/details/79268902