Python语法知识点
1.can’t multiply sequence by non-int of type ‘float’
2.UnicodeDecodeError: ‘gbk’ codec can’t decode byte 0xb3 in position 2: incomplete multibyte sequence
在使用python处理坐标的时候,需要将字符串根据度分秒中的秒先分割,然后在处理,在使用split函数的时候,我遇到了这个错误。字符串坐标如下
1 | 1.28°12′09.090″121°09′16.756″ 2.28°12′26.701″121°09′41.847″ 3.28°12′33.649″121°09′35.792″ 4.28°12′20.931″121°09′18.179″ 5.28°12′28.951″121°09′07.653″ 6.28°12′45.098″121°09′06.679″ 7.28°12′44.782″121°08′56.026″ 8.28°12′27.097″121°08′56.605″ |
其中的度分秒都是中文字符串,这个使用用split分割函数,对数据进行分割,会出现:“UnicodeDecodeError: ‘gbk’ codec can’t decode byte 0xb3 in position 2: incomplete multibyte sequence”错误。
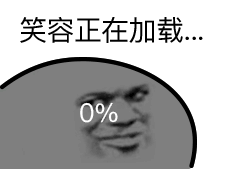
这个时候,要将字符串先进行utf8编码,然后在进行调用split函数,就可以了。
完整代码
1 | #!/usr/bin/python |
主要是encode函数。
3.判断对象是否为空
1 | if x is None: |
1.python判断对象是否为None
4.遍历字典
1 | ## 遍历key |
1.Python: 遍历字典
5.int和string相互转换
1 | ## 10进制string转化为int |
1.Python int与string之间的转化
6.用户输入
使用while True,循环等待用户输入,当用户输入 确定按键的时候,退出循环。
1 | ## 进行验证 |
7.路径拼接
这里有一个坑,就是第二个参数不能带斜杠,否则就会忽略第一个参数
1 | # 无效 |
【1】.python os.path.join()拼接不成功,有个坑 使用os.path.join第二个参数的首个字符如果是”/“ , 拼接出来的路径会不包含第一个参数
【2】.使用os.path.join无效/不起作用的原因 os.path.join(a,b)在以下情况下会起不到连接作用,并返回b:a,b中存在一个为绝对路径,即不是patha/pathb的形式,而是/patha/pathb的形式