Spring Boot从二到三
继续爬坑。
1.spring boot的@RequestBody获取前端传入的字符串
1 |
|
本意是想将前端传入的json字符串,直接转换为inspection类。前端传入的json字符串如下:
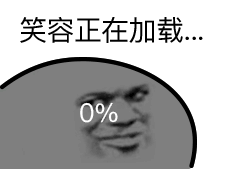
结果还是报错:org.springframework.http.converter.HttpMessageNotReadableException: Required request body is missing
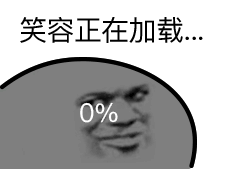
经过测试,要想用requestbody,必须使用post方式,下面这样就可以了。
1 | $.ajax({ |
1.spring-boot前后端利用jquery进行ajax通信(以json为媒介)
2.@RequestBody对象为空,异常Required request body is missing错误解决
3.Springboot之接收json字符串的两种方式-yellowcong
4.spring boot get和post请求,以及requestbody为json串时候的处理
5.SpringBoot 中 @RequestBody的正确使用方法
6.Spring之RequestBody的使用姿势小结 (这个总结其实挺全面的,讲了@RequestBody和@RequestParam等的用法)
另一个问题是,在请求时,出现了这么一个错误: Unrecognized token ‘id’: was expecting (‘true’, ‘false’ or ‘null’); nested exception is com.fasterxml.jackson.core.JsonParseException: Unrecognized token ‘id’: was expecting (‘true’, ‘false’ or ‘null’)
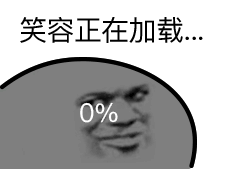
解决方法是对要传递的对象数据进行 JSON.stringify(inspectionObj),这样在使用时就没有问题了。最后的ajax如下:
1 | $.ajax({ |
1.springmvc与ajax交互常见问题
2.SpringBoot命令行(CommandLineRunner初始化启动)
SpringBoot除了能开发web应用程序的功能外,还可以开放命令行工具,比如我想在应用程序启动的时候,就执行某些操作,但是直接在main函数中,调用某个bean的方法,会提示通过@Autowired自动注入的bean是一个空值。
1 |
|
这里的clickhouseImport就会是一个空。如果想实现在应用程序启动之后完成一些初始化工作,就需要实现CommandLineRunner接口,然后重写run方法。这样就既可以进行初始化,又可以使用SpringBoot提供的各种bean服务了,比如@Service等。当然也可以在main函数之外实现一个单独的@Component实现CommandLineRunner接口,SpringBoot依然可以实现初始化。
1 |
|
1.Spring Boot 启动加载数据 CommandLineRunner
2.Spring boot 启动之后,执行某些初始化的几种方法
3.SpringBoot之CommandLineRunner接口和ApplicationRunner接口
4.Bean装配,从Spring到Spring Boot
5.用 SpringBoot 实现一个命令行应用
3.读写Resource文件夹的文件
我想读取resource文件夹下的insert.sql文件
【尝试方法】
(1) 方法一:使用ResourceUtils类进行读取
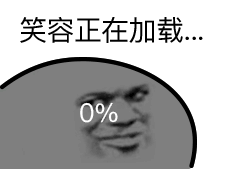
但是使用”classpath:insert.sql”,出现了无法找到文件的问题
1 | ResourceUtils.getFile("classpath:insert.sql"); |
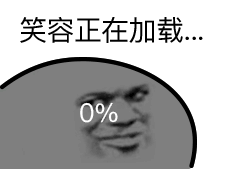
(2) 方法二:直接将”classpath:insert.sql”作为参数传递个FileOutputStream,结果也没有引用到resource文件夹下的insert.sql文件,但是没有报错,结果不知道写到哪里去了。
1 | public static void fileAppend(String file, String conent) { |
(3) 方法三:我也尝试了使用google的guava进行操作,但是就是无法获取resource文件夹下的文件。如下内容,使用getResource无法获取resources/test文件夹下的insert.sql文件,显示NotFound。
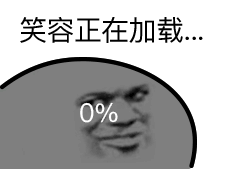
1.springboot读取resource下的文本文件 使用的是ResourceUtils这个类
2.Spring Boot 获取 java resources 下文件 有两种方式,第一种也是使用的ResourceUtils这个类,第二种使用的是ClassPathResource
3.Guava(Resources用法) 读取 classpath 中的文件、读取HTTP 这里提到了借助Guava库的Resource类进行文件的读写
5.java读取resource目录下文件的方法示例 和上面的一样,也是使用了Guava的Resource类
6.java中outputStream与inputStream的相互转换
7.Java – Write an InputStream to a File
8.Java读取resource文件/路径的几种方式
9.java读取resource目录下的文件
10.getResource方法路径参数的不同
(4) 方法四:最后还是没有解决读取到的target\classes文件下的文件而不是resources文件夹的文件
1 | /** |
1.在Spring Boot快捷地读取文件内容的若干种方式
2.SpringBoot读取Resource下文件的几种方式 有四种方式读取Resource下文件
3.SpringBoot读取Resource下文件的几种方式 和上面的一模一样
【解决方法】
使用SpringBoot的ClassPathResource,读取resource目录下的inp/pipeline.inp,注意引用的方法。
1 | ClassPathResource classPathResource = new ClassPathResource(inpFilePath+"pipeline.inp"); |
1.SpringBoot读取Resource下文件的几种方式 第一种:ClassPathResource加上classPathResource.getInputStream();第二种:Thread.currentThread().getContextClassLoader().getResourceAsStream;第三种:this.getClass().getResourceAsStream;第四种:ResourceUtils.getFile。前三种方法在开发环境(IDE中)和生产环境(linux部署成jar包)都可以读取到,第四种只有开发环境 时可以读取到,生产环境读取失败。这几个方法在其他的文章中也看到过。
2.Java 按行读取文件